Hope you got your thinking cap on before you start this lesson. We are going to dive into Python Classes. If you are going to be writing a lot of code and reusing most of it.
Then Python Classes is going to work wonders for you. This will begin your understanding of code structure. Additionally, you will want to think about your code structure before you write any code. This will minimize redoing code when your script gets bigger.
This topic is part of our Introduction to Python Course. (It’s Free). We like to keep things simple and leverage Google’s Colab which allows you to write python right in your browser. You can follow along with our notebook if you would like. If you want more details on this topic you can visit Python.org.
Topics Covered
What is a Python Classes?
A Python Class is a defined blueprint or prototype created by the coder. Classes allow you to bundle data, functions, methods together. This is what makes Python an Object-Oreintated Program.
Create a Class
Creating Python Classes creates an object, allowing you to reuse what code is inside the class. This becomes very useful when writing large amounts of code. Along with being able to reuse your functions and methods for different parts of your code.
To create a class you simply type the word class and the name you want to give the class. Furthermore make sure you name the class to something that the code does. This will help you later down the road when you need to change or add to that class.
Second you will follow that name with a colon to seal the deal. Lastly below the class name is the body. This is where all the good stuff will go. Altogether you will need to have something in this body.
You can start off by using the pass statement if you have no code starting off. To demonstrate this process I will create a simple class and print what is in the variable below.
class exampleStudents:
pass
class exampleStudents:
numStudents = 5
print(exampleStudents.numStudents)
5
As you can see, in order to get the variable to print we call in like a method. If you don’t know what methods are, check out our post on Python Functions. In that post we talk about using functions that also allow repeat code use. A Python method is like a Python function, but it must be called on an object. And to create it, you must put it inside your class.
Class Object
So your question might be, “Why do we need to make a class an object?”. To that question the simplest answer can be, it allows you to shorten your code. Making a class into an object is as simple as creating the class. You can take a variable that can associate to the class and have it equal the class.
oldStudents = exampleStudents()
print(oldStudents.numStudents)
5
This creates a proper object of the class in Python for you to independently change and reuse. Not to mention you can now create many objects of the same class. Allowing you to change the variables in the class without affecting the original class.
newStudents = exampleStudents()
newStudents.numStudents = 2
oldStudents.numStudents = 7
print(oldStudents.numStudents)
print(newStudents.numStudents)
print(exampleStudents.numStudents)
7
2
5
In this example you can see that we created two class objects of the Students Class. We then change the variable within the class and see that they all have different values.
Class Functions
To start this section we will add a function into our previous class, Students. To keep this simple we will add a function that prints a string. Remember to check out the Functions post link above if you don’t know about functions. The new function in the class would look like the example below.
class countStudents:
numStudents = 5
def countStudents(self):
print("We have blank students in our class.")
When you add functions in your class it is best to use self as the first parameter. We will go over why later in this course. For now you can see we print a string in the class. Now lets access that function and print the string in the class.
newStudents = countStudents()
newStudents.countStudents()
We have blank students in our class.
As you can see we use the newStudents object class to call the countStudents(). This runs and prints the message within the function in the class. Similarly this function was called just like the variable. The only difference is the parenthesis where the function parameters can go. Now this is only scratching the surface of what classes can do.
self
Parameter
Before we get into Methods let’s talk about the self
parameter. The first parameter is a reference to your class instance, and has to be used to access the variables that it belongs to.
Python makes the instance to which the method belongs to be passed automatically, but not received automatically. The first parameter of your function does not have to be named self, you can call it whatever you like. In most cases it’s just better to call it self for better code readability.
That is why I will use self as the first parameter in my examples, because I know that it belongs to that method. Here is an example of naming the first parameter something different in the function.
class crazyStudents:
def countStudents(count):
print("There are 7 students and one is named Frank.")
def nameStudents(somthingCrazy):
print("Print something crazy!")
oldStudents = crazyStudents()
oldStudents.nameStudents()
Print something crazy!
Methods In Classes
Another big function involving the class is the __init__ constructor. All classes have this method, which is always executed when the class is being initiated. You will use the __init__ constructor to give values to your class properties when it is created.
You may also hear others refer to the double underscore as “dunder”. Now you might be wondering what’s the difference between function and method. Well the difference is that a method is associated with an object, while a function is not. Let’s see how that would look in the following example.
class Students:
def __init__(self, numStudents, nameStudent):
self.numStudents = numStudents
self.nameStudent = nameStudent
def countStudents(self):
print(f"There are {self.numStudents} students and one is named {self.nameStudent}.")
oldStudents = Students(7,"Frank")
oldStudents.countStudents()
There are 7 students and one is named Frank.
Does that blow your mind? Hope you had your thinking cap on. Now imagine if those were not hard coded values we put in the object class. Can you see the endless possibilities?
With that being said, the example shows that we initiated the variables for the class. Then created a countStudents method and used the variables we made. We then made an object class and gave the hard code values.
Keep in mind that there are a lot more methods that you can use. I will do another post on class methods, so stay tuned. For now you can check out Python.org for more class methods.
Modify Classes
Here we will go over a few ways to modify code in your class. The first one modifier I already showed an example. It’s where we changed the numStudents variable. Second one is deleting the variable. Lastly is deleting an object that you created from the class.
I will give examples of all three below. I will start with my class with my initiated variables and my countStudent method.
class modifyStudents:
def __init__(self, numStudents, nameStudent):
self.numStudents = numStudents
self.nameStudent = nameStudent
def countStudents(self):
print(f"There are {self.numStudents} students and one is named {self.nameStudent}.")
oldStudents = modifyStudents(7,"Frank")
print(oldStudents.numStudents)
print(oldStudents.nameStudent)
7
Frank
oldStudents.numStudents = 8
oldStudents.numStudents
8
print(vars(oldStudents))
del oldStudents.nameStudent
print(vars(oldStudents))
{'numStudents': 8, 'nameStudent': 'Frank'}
{'numStudents': 8}
print(oldStudents)
del oldStudents
<__main__.modifyStudents object at 0x7f16d10830d0>
Cheat Sheet Python Classes
Syntax
class ClassName:
def __init__(self, parameters):
self.parameters = parameters
def methodName(self):
method body
Common Methods
- object = class: Create Object Oreintated Class
- object.variable: Pull variable from class
- object.methodName(): Run method from class
- vars(object): Shows variables for object class
- del object.variable: This will delete the variable
- del object: This will delete the object
- __init__(): The __init__() function assigns values to object properties
Common Mistakes
- Error Handling-If your class will be empty temporally it will need the pass statement
- Incorrect Indentation-Don’t forget to indent after creating methods
- Misusing The __init__ Method-This is used to initiate your class with values, you should try to return any values.
- Class Variables-Python uses MRO which is the order in which Python searches.
- Python Standard Library Module Names-Careful not to use Python keywords or modules for variables
- Specifying Wrong Parameters In Class-Don’t forget what type() your arguments should be for you parameters.
Typical Use
Python Classes allow you to bundle data, functions, methods together. Making it a great option to reuse repeat code throughout your script.
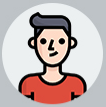
I have my Google IT Support Professional Certificate, and Google IT Automation with Python Specialization Certificate. I continue to develop my skills in networking, operating systems, system administration, troubleshooting, communication, security, and programming. I train constantly through computer courses that include 80% hands-on practice. I love learning new technical skills, analytical skills, and programming through Udemy, Coursera, and any other sources every day. I have 10 years of computer skills and 2 years of programming with Python, C#, JavaScript, and HTML.