Python String Formatting Operation is the standard way for Python to store characters as data used in variables. This string data can be formatted in endless ways from capitalizing letters to turning integers into a string.
In this course, we will go over string formatting and the operators that can be used with strings. So what makes string formatting amazing is you can take user input and format it to what you need. Let’s say you have a script that takes a user’s input, though you don’t want user names to be capital letters.
Now, for instance, you can use operators to compare if the user puts in numbers in that username. So with all that being said, let’s begin Our Journey Into Python.
This topic is part of our Introduction to Python Course. (It’s Free). We like to keep things simple and leverage Google’s Colab which allows you to write python right in your browser. You can follow along with our notebook if you would like. If you want more details on this topic you can visit Python.org.
Topics Covered
What is String Formatting Operation?
Python String Formatting Operation is the use of methods to change the structure of that specific string. Therefore, this can also be applied to turning non-strings like numbers into strings.
Format Strings
Formatting strings has a wide range of options, but here we will cover the most commonly used ways of formatting. Here we will learn how to add strings to strings with other data types. First, we will go over the different ways to change strings. Now concatenation is the simplest way of adding one string to another.
firstName = "John"
lastName = "Schmidt"
print(firstName + " Jacob Jingleheimer " + lastName)
John Jacob Jingleheimer Schmidt
Secondly, the way you can format is use .format(), which uses index numbers to place them where you need them. You can also use placeholder names but that can be unnecessary and can get lengthy.
print("Welcome to {}" .format("'Journey Into Python'"))
print("Welcome to {}, your go-to {}".format("'Journey Into Python'","learning blog!!!"))
print("This is the order {}, {}, {}, ect...".format(1,2,3))
Welcome to 'Journey Into Python'
Welcome to 'Journey Into Python', your go-to learning blog!!!
This is the order 1, 2, 3, ect...
print("{1} {3} {2} {0}.".format("indexing","This","using","is"))
print("{one} {two} {three} {four}.".format(four="naming",one="This",three="using",two="is"))
This is using indexing.
This is using naming.
String Methods
You’ll notice that we used the .format()
to change or manipulate a string. This is one of Python’s methods for strings. Now we will move on to the power of other methods for strings. I will go over a few but if you want to learn about other methods for strings, check out Python’s String Methods.
The first method to go over is the .upper()
and .lower()
to change text size. This also does not change the variable just creates a new instance with the change.
userName = "Hello World!"
print(userName.upper())
print(userName.lower())
print(userName)
HELLO WORLD!
hello world!
Hello World!
Another common use method is .strip()
, this will remove any whitespace around the string. This comes in handy with user input and they put a space before or after the string.
stripString = " Hello World! "
print(f"{stripString} is {len(stripString)} characters long.")
print(f"{stripString.strip()} is now only {len(stripString.strip())} characters long.")
Hello World! is 22 characters long.
Hello World! is now only 12 characters long.
The .replace()
takes two arguments, what you want to be replaced and the replace with. This works great for changing over a companies email address.
email = "john@example.com"
print(email.replace("@example.com","@newemail.org"))
john@newemail.org
Last but not all, the .split()
a method that will take a string of words with a pre-defined separator and turn it into a list. Remember that this does not change the variable, to do that you will have to make it a new variable.
names = "Tom, Jerry, Bugs Bunny, Road Runner, Daffy, Elmer"
print(names, type(names))
print(names.split(","))
splitNames = names.split(",")
print(type(splitNames), splitNames)
Tom, Jerry, Bugs Bunny, Road Runner, Daffy, Elmer <class 'str'="">
['Tom', ' Jerry', ' Bugs Bunny', ' Road Runner', ' Daffy', ' Elmer']
<class 'list'=""> ['Tom', ' Jerry', ' Bugs Bunny', ' Road Runner', ' Daffy', ' Elmer']
More on Formatting
A non-string type can not be added to a string, like an integer. But you can either change it into a string or use the .format
one that will do it for you. Now let’s say you get user input and you don’t know what they will enter, but you want that input displayed in your text.
userInput = 99
printOutput = "I am " + userInput + "% sure, I am learning to code."
print(printOutput)
TypeError: can only concatenate str (not "int") to str
userInput = 99
printOutput = "I am "+ str(userInput) +"% sure, I am learning to code."
print(printOutput.format(userInput))
userInput = 99
printOutput = "I am {}% sure, I am learning to code."
print(printOutput.format(userInput))
I am 99% sure, I am learning to code.
I am 99% sure, I am learning to code.
A new format that was implemented in Python 3.6 is the f-string format. This can make simple work out of inputting variables in strings. Also makes it very easy to read by anybody.
name = "Python"
age = 30
print(f"Hello {name}, you are {age} years old.")
Hello Python, you are 30 years old.
Here is the example from the intro, where we take input and make it all lowercase. It is then passed through some if statements to check the length and if there are any numbers. Now, this isn’t logical but you get the idea of what can be done with strings and how you can put that new knowledge to use.
myInput = input("Enter Password: ")
myInput = myInput.lower()
if len(myInput) < 8:
print(f"'{myInput}' needs to be more than 8 characters long.")
elif myInput.isalpha() == False:
print(f"'{myInput}' can not have numbers in it.")
else:
print(f"Your '{myInput}' has been accepted!")
Enter Password: ImL0v3N1t
'iml0v3n1t' can not have numbers in it.
Enter Password: PswD
'pswd' needs to be more than 8 characters long.
Enter Password: Password
Your 'password' has been accepted!
You can also format variables using Pythons format operations. Link to Common String Operations in Python.
print("{:*^30}".format("centered"))
print("{:<30}".format("left aligned"))
print("{:>30}".format("right aligned"))
***********centered***********
left aligned
right aligned
Operators
Operators are mathematical symbols and logic used to evaluate elements in python. These are things like +, -, =, and, or, not. With these operators, you are able to create decisions within Python.
Simple use would be is 1 < 2 return true or false. Then you can have Python execute more code depending upon the return value. Here is a list of Python’s data type Operators. The first example shows the value of each letter then compares the alphabet value in the two strings.
stringValue1 = [ ord(x) for x in "Penny" ]
print('Penny:', stringValue1)
stingValue2 = [ ord(x) for x in "Paul" ]
print('Paul:', stingValue2)
if "Penny" > "Paul":
print("Penny has larger alphabet value.")
elif "Penny" < "Paul":
print("Paul has smaller alphabet value.")
Penny: [80, 101, 110, 110, 121]
Paul: [80, 97, 117, 108]
Penny has larger alphabet value.
Here is an example from another course List of Lists. Now= is used for the count, < to compare the count to length, += to add one to count, and in is used with the for statement. For a list of comparison operators, check out Python Documents at this link
nestedList = [["list0index0","list0index1"],["list1index0","list1index1"]]
count = 0
while count < len(nestedList):
for i in nestedList:
print(f"Index number is {count}")
print(i)
count += 1
Index number is 0
['list0index0', 'list0index1']
Index number is 1
['list1index0', 'list1index1']
Cheat Sheet
String Formatting:
String formatting is the use of methods to change the structure of that specific string. This can also be applied to turning non-strings like numbers into strings.
Syntax
x = "string's"
or x = '"string"'
Common Mistakes
Adding integers to strings without converting them to strings format first.
String methods do not change the original string, only show the change. To implement the change you need to make newstring = oldstring.method().
Triple check your syntax when adding strings with other strings. Don’t forget strings need to be in quotes, double quotes if you need to use apostrophes, and single quotes if you use double.
Operators and Methods for String’s
:0<
Shifts “string” to the left dependent on number:0>
Shifts “string” to the right dependent on number:0^
Makes your “string” centered dependent on numberand
Returns True if both statements are true “string” == “string” and “string” == “string”or
Returns True if one of the statements is true “string” == “string” or “string” == “string”not
Reverse the result, returns False if the result is true not(“string” == “string” and “string” = “string”)is
Will Return True if both variables are the same object “string” is “string”is not
Makes it True if both variables are not the same object “string” is not “string”in
Returns True if a sequence with the specified value is present in the object “string” in “string”not in
Returns True if a sequence with the specified value is not present in the object “string” not in “string”.upper() .lower()
Changes size of text in string.strip()
Remove empty space around string.split('separator')
Change long string with same separators into a list.replace('what','to')
Replace string with another string, can be one character or entire string
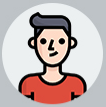
I have my Google IT Support Professional Certificate, and Google IT Automation with Python Specialization Certificate. I continue to develop my skills in networking, operating systems, system administration, troubleshooting, communication, security, and programming. I train constantly through computer courses that include 80% hands-on practice. I love learning new technical skills, analytical skills, and programming through Udemy, Coursera, and any other sources every day. I have 10 years of computer skills and 2 years of programming with Python, C#, JavaScript, and HTML.