Let’s get that brain going and learn more about Python and how to use Python functions. You ever create a little code here and a little code there, then think, “wish I could reuse that code.” Well, you’re in the right place because functions do exactly that.
Python is like an office building, and functions are like all those works in a cubicle. They are all by themselves, just waiting to be called so they can all start working together when they are needed. So let’s start your journey into python and learn how to create those workers to use them when you need them.
This topic is part of our Introduction to Python Course. (It’s Free). We like to keep things simple and leverage Google’s Colab which allows you to write python right in your browser. You can follow along with our notebook if you would like. If you want more details on this topic you can visit Python.org.
Topics Covered
What are Python Functions?
A Python function is a block of code that will only work when you call it somewhere in your code. Functions can be used multiple times within the same script. Functions can take values, known as parameters, that can be used inside that block of code where you can return elements to use elsewhere in your script.
How To Create A Python Function
I keep using the word function, making me think of that catchy song. Function, Junction, what’s your Conjunction by Schoolhouse Rock. Back to Python, to create a function, you first have to define that you’re making a block of code a Python function.
If you guessed the keyword def, you would be correct. Then you will give your Python function a name, best to use what the function does, with no spaces. Now at this time, you will follow that with parentheses and a colon. The parentheses are where your parameters will go, and we’ll give more details later. So let’s give that a try.
def questionString():
print("Why won't this print?")
print("Because I don't work till called")
return
You will notice after you have created your Python function, if your following along, is that Python functions can also be folded in most IDE’s. Also, if you tried to run the code, you will notice it didn’t print anything. That’s because we created the function. Now we have to call the function for it to run the code inside.
How To Call A Python Function
Well, we thought we should draw this out and make it elaborate, but let’s get to it. We call the Python function by simply typing the name of the function. Oh, and don’t forget your parentheses when you call it. Read my warning below if you’re testing and you get the NameError:
*Watch Out*
Now, something to keep in mind when creating functions is they need to be created before you can call them in your code. So you can’t call your function at the top of your code then create it at the end. You will get that big ol’ NameError: name is not defined.
The cool thing about Colab, which uses Jupyter, is you can run the cell with your defined function and then call your function anywhere.
questionString()
Why won't this print?
Because I don't work till called
How Python Function Parameters Work
In this objective, we will show the basics and then come back at the end of the lesson with details. I don’t want to melt your brains only three objectives in, we want you to get the basics first.
We talked earlier about the parentheses, and you can put parameters there to use in your function. Since functions are like a little block of a program, you can make your own variables there. These variables in your function are not affected by what’s outside, but the variables you define outside can be used inside the function.
Make sense, or did we lose you, I think I lost myself a little. I hope an example can explain it better. If not, let me know if you need more clarification in the comments section below.
var1 = "Outside Function"
print(var1)
def variableFunction():
var2 = "Inside Function"
print(var1, var2)
# print(var2)
# will not work, remove hashtag to try it out
variableFunction()
Outside Function
Outside Function Inside Function
def useParameters(strValue):
print(f"Here a {strValue}, There a {strValue}, Everywhere a {strValue}.")
useParameters("Pig")
useParameters("Cow")
useParameters("Chicken")
Here a Pig, There a Pig, Everywhere a Pig.
Here a Cow, There a Cow, Everywhere a Cow.
Here a Chicken, There a Chicken, Everywhere a Chicken.
Does that remind you of MadLibs, where you fill in words, and it ends up making a funny story? You could totally do that with a function! Functions can have as many parameters as you want. Just make sure that when you call your function that all the arguments have a value, or you will get the big ol’ TypeError: missing argument.
I want to mention this too, a parameter is the variable listed inside the parentheses in the function definition. An argument is a value that is sent to the function when it is called. This can be interchangeable between people, but to a function that is the correct use. We’ll come back to this later…
How To Use Return In Python Function
First, what is a return value? Well, since functions are their own little thing. You don’t always want to print the value you create in the function.
So Python has made it so you can get return values from a function with the keyword return. To accomplish this, you type return at the end of your function and the value that you want the function to return.
This can be an answer to a math problem or some crazy formulation that iterates through a dictionary and returns all the keys. Can check more on Dictionaries Here. I will keep it simple, but you can build on this example.
def mathProblem(x):
return 20 * x
mathProblem(20)
400
food = "turkey" # input("Pick A Food: ")
things = "stockings" # input("Pick a Thing(Plural): ")
action = "jump" # input("Pick an Action Verb: ")
def madLib(food, things, action):
lib1 = print(f"There once was a gingerbread man who had two {things} for eyes and a {food} for a nose.\n"
f" He always said, '{action.capitalize()} as fast as you can, you can't catch me, I'm the gingerbread man.'\n")
lib2 = print(f"I saw this person eating a {food}.\n"
f" I {action}ed over there and asked, 'What {food} {things} do you like?'\n")
return lib1, lib2
print(madLib(food, things, action))
There once was a gingerbread man who had two stockings for eyes and a turkey for a nose.
He always said, 'Jump as fast as you can, you can't catch me, I'm the gingerbread man.'
I saw this person eating a turkey.
I jumped over there and asked, 'What turkey stockings do you like?'
(None, None)
As you can see, we typed out print() with our function name and then gave it the arguments it needed; these are passed into the function parameters as variables. From there, the function runs through the code, and we ask for a return on the variables we created in the function.
We get a (None, None) because the Madlib function is just performing an action, not calculating values. One thing we didn’t mention is that instead of using return you can use pass if your function is empty. This is handy if you are testing code and your function will be empty for the moment.
What Parameters Can Python Functions Use
When creating functions, you can use different kinds of parameters. The first is a regular argument and having more than one.
def oneParameter(x):
return x
print(oneParameter(12))
def manyParameter(x,y,z):
return x,y,z
print(manyParameter(10,2,34))
12
(10, 2, 34)
The second would be using an asterisk with the variable. This is used if you do not know how many parameters you will need.
def asterisk(*x):
return x
print(asterisk(1,2,3,4,5,6))
(1, 2, 3, 4, 5, 6)
The next parameter would be using keywords to define the different arguments.
def keyword(x,y,z):
return x,y,z
print(keyword(y=10,z=36,x=15))
(15, 10, 36)
You can then add a double asterisk along with keywords if you don’t know how many arguments the keyword might have.
def asteriskKeyword(**x):
return x["xz"],x["xy"]
print(asteriskKeyword(xx=56,xy=10,xz=34,yy=54))
(34, 10)
Another good parameter, which is also good coding practice, is giving your parameter a default value. Giving your parameter a default value makes it an optional argument. This works well because there is no need to specify a value for an optional argument when you call a function. This will keep your script from giving an error if an argument is not given because the default value will be used.
def defaultKey(name="N\A",age="N\A"):
x = f"Hello {name}, your age is {age}."
return x
print(defaultKey())
print(defaultKey("Jack"))
print(defaultKey(age="23"))
print(defaultKey("Jane","36"))
Hello N\A, your age is N\A.
Hello Jack, your age is N\A.
Hello N\A, your age is 23.
Hello Jane, your age is 36.
You can use any data type for an argument in a function parameter.
fruitsList = ["orange", "plum", "mango"]
def foodFunction(food):
for x in food:
print(x)
foodFunction(fruitsList)
orange
plum
mango
What Is and How To Use Recursion
Recursion is, in its basic form, where you call your function in your function. You should be very careful with recursion as it can be very easy for a function to never terminate or one that uses excess amounts of memory or processor power. Though, when written carefully, recursion can be very efficient. We will go into more details on recursion in another post.
def recursion(x):
if x > 0:
print(recursion(x-1))
else:
x = 0
return x
recursion(2)
0
1
2
Cheat Sheet Python Functions
Syntax
def function_name():
code block
return
Parameters
function_name(parameter):
When you require an argument*args
Unknown Arguments (variable= x, y, z)kwargs
Keyword Arguments (x= “x”, y= “y”)**kwargs
Unknown Keyword Arguments (x= x[x, y, z])argument = "default"
No Argument Uses Default Argument
Global vs. Local variables
Variables defined inside a function body are local variables, and those defined outside are global variables.
This means variables in your function are not affected by what’s outside, but the variables you define outside can be used inside the function. When you call a function, the variables declared inside the function are local variables.
Common Mistakes
Calling a function before the function is defined
A function with parameters not getting the required arguments
A function does not execute code block till called
Typical use
To create reusable code blocks that streamline coding. These functions are called user-defined functions.
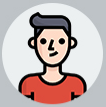
I have my Google IT Support Professional Certificate, and Google IT Automation with Python Specialization Certificate. I continue to develop my skills in networking, operating systems, system administration, troubleshooting, communication, security, and programming. I train constantly through computer courses that include 80% hands-on practice. I love learning new technical skills, analytical skills, and programming through Udemy, Coursera, and any other sources every day. I have 10 years of computer skills and 2 years of programming with Python, C#, JavaScript, and HTML.