Python has a few different data types that are used to group other values together, to clarify Python has six standard data types. Therefore that makes lists a versatile data type. These lists can be written as a list of comma-separated elements between square brackets.
A Python list can contain elements of different types but usually, the elements all have the same type. You Journey Into Python will begin with understanding what a python list is and then learn the different uses of lists and how to apply them.
You ever have a bunch of math problems and wanted to store the answers and then needed to use those answers to answer another problem? In conclusion, you will have all the answers you need.
This topic is part of our Introduction to Python Course. (It’s Free). We like to keep things simple and leverage Google’s Colab which allows you to write python right in your browser. You can follow along with our notebook if you would like. If you want more details on this topic you can visit Python.org.
Topics Covered
What is a List?
Lists are used to store multiple elements in a single variable. Also lists are one of 4 built-in data types in Python used to store collections of data. A Python list is created using square brackets []
with elements that are ordered, changeable, and allow duplicate values. List elements are indexed, where the first element will have an index of [0], the second element with index [1], etc.
Python Lists
If we add any new elements to a list, the new elements will always be placed at the end of the list, unless you specify where you want the element to go. Most importantly lists are mutable, meaning that we can change, add, and remove elements in a list after it has been created. Lists can also have elements of any data type.
exampleList = ["apple", 1, None, True]
print(exampleList)
['apple', 1, None, True]
New List
New lists can be created in two ways, but always has to be defined before changing. You can make an empty list or a list with values. Once you have defined a list you can add, remove, or change in many different ways.
emptyList = []
print(emptyList)
thisList = ["add", "remove", "change"]
print(thisList)
[]
['add', 'remove', 'change']
You can add to a list several different ways, by using the .insert()
or .append()
you can add a single element or a list of elements. You can use .extend()
to add an existing list.
addList = ["Stuff", "More Stuff"]
print(addList)
addList.insert(2, "A lof of Stuff")
print(addList)
addList.append("Stuff at the end")
print(addList)
# Can add any kind of data type with .extend()
# and it will be converted to list syntax, dictionary will only convert keys
addTuple = ("Tuple Stuff", "More Tuple Stuff")
addList.extend(addTuple)
print(addList)
['Stuff', 'More Stuff']
['Stuff', 'More Stuff', 'A lof of Stuff']
['Stuff', 'More Stuff', 'A lof of Stuff', 'Stuff at the end']
['Stuff', 'More Stuff', 'A lof of Stuff', 'Stuff at the end', 'Tuple Stuff', 'More Tuple Stuff']
Removing data can be accomplished by using these functions:
print(addList)
addList.remove("Stuff")
print(addList)
addList.pop(2)
print(addList)
# del with no index will delete list
del addList[1]
print(addList)
addList.clear()
print(addList)
['Stuff', 'More Stuff', 'A lof of Stuff', 'Stuff at the end', 'Tuple Stuff', 'More Tuple Stuff']
['More Stuff', 'A lof of Stuff', 'Stuff at the end', 'Tuple Stuff', 'More Tuple Stuff']
['More Stuff', 'A lof of Stuff', 'Tuple Stuff', 'More Tuple Stuff']
['More Stuff', 'Tuple Stuff', 'More Tuple Stuff']
[]
Changing data in a list is just as simple as adding, with a few differences.
changeList = ["Things","I","Change"]
print(changeList)
changeList[1] = "We"
print(changeList)
# Here I changed "We" with ("I","Want","To") and "Change" moves accordingly
changeList[1:2] = ["I","Want","To"]
print(changeList)
changeList.insert(4, "Have")
print(changeList)
['Things', 'I', 'Change']
['Things', 'We', 'Change']
['Things', 'I', 'Want', 'To', 'Change']
['Things', 'I', 'Want', 'To', 'Have', 'Change']
Nested Lists
Nested lists can look overwhelming but keep in mind that a list in a list is still going to have an index. So if you have a nested list you would just access it by its index like so:
nestedList = [["list0index0","list0index1"],["list1index0","list1index1"]]
print(nestedList[1][0])
list1index0
If you don’t know how many lists are in a list you can use the len()
function to find out how many lists there are. You can also use a for loop to go through the list to pull each list of lists. Both of those can be used when you don’t know what’s in the list of lists with a while loop.
print(len(nestedList))
for i in nestedList:
print(i)
2
['list0index0', 'list0index1']
['list1index0', 'list1index1']
count = 0
while count < len(nestedList):
for i in nestedList:
print(f"Index number is {count}")
print(i)
count += 1
Index number is 0
['list0index0', 'list0index1']
Index number is 1
['list1index0', 'list1index1']
List of Lists
Creating a list of lists can be done by using the functions mentioned above in New Lists [adding lists]. You can use the .append()
function to add more and more to a list, whether it is to add one element or another list of elements.
In the example below we create an empty list, then in the first for loop append two empty lists. The second for loop takes a range and appends that to each empty list.
lists = []
for i in range(2):
lists.append([])
for j in range(0,12,2):
lists[i].append(j)
print(lists)
[[0, 2, 4, 6, 8, 10], [0, 2, 4, 6, 8, 10]]
List Comprehension
You can create lists with a for loop, but there’s a simpler way to do this, list comprehension. List comprehensions allow you to create a new list from a single line. This is just like saying for number in number(range) multiple numbers by 2.
The next one is for elements in thisList add an element if it is equal to an element. Careful creating list comprehension as they can begin to get confusing for someone to read or for you a year later.
comList = [number*2 for number in range(1,11)]
print(comList)
#Can also use if statements with comprehesion
comList2 = [element for element in comList if element >= 12]
print(comList2)
[2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
[12, 14, 16, 18, 20]
Flatten List of Lists
When you have a list of lists and you need to combine them all into one list you can loop through the list of elements and put them in a new single list. Below we create an empty list, then iterate through the loop to get all of the elements of the list of lists.
Finally, we add all those elements to a new list in the order they were in the list.
lists = [[0, 2, 4, 6, 8, 10], [0, 2, 4, 6, 8, 10]]
flattenList = []
for insideList in lists:
for elements in insideList:
flattenList.append(elements)
print(flattenList)
[0, 2, 4, 6, 8, 10, 0, 2, 4, 6, 8, 10]
Cheat Sheet Python List:
Friendly link to Cheat Sheet Page
Python List
Lists are mutable, storing multiple elements in a single variable. Lists are one of 4 built-in data types in Python used to store collections of data.
Syntax
list = ["element","ect..."]
Common Mistakes
Not initializing an empty list before manipulating elements within a list.
Syntax for list missing or misplaced. ["",""]
Trying to index an element that is not in the list.
Method | Description |
---|---|
list[i] = x | Replaces the element at index i with x |
list.append(x) | Inserts x at the end of the list |
list.insert(i, x) | Inserts x at index i |
list.pop(i) | Returns the element a index i, also removing it from the list. If i is omitted, the last element is returned and removed. |
list.remove(x) | Removes the first occurrence of x in the list |
list.sort() | Sorts the items in the list |
list.reverse() | Reverses the order of items of the list |
list.clear() | Removes all the items of the list |
list.copy() | Creates a copy of the list |
list.extend(other_list) | Appends all the elements of other_list at the end of list |
Check out the official documentation on Python.org.
List comprehension
[expression for variable in list] Creates a new list based on the given list. Each element is the result of the given expression.
[expression for variable in list if condition] Creates a new list based on the given list. Each element is the result of the given expression; elements only get added if the condition is true.
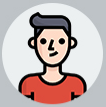
I have my Google IT Support Professional Certificate, and Google IT Automation with Python Specialization Certificate. I continue to develop my skills in networking, operating systems, system administration, troubleshooting, communication, security, and programming. I train constantly through computer courses that include 80% hands-on practice. I love learning new technical skills, analytical skills, and programming through Udemy, Coursera, and any other sources every day. I have 10 years of computer skills and 2 years of programming with Python, C#, JavaScript, and HTML.