You have come to the right place to learn about python operators, no I’m not talking about people who operate equipment. You’re here to learn about Python Operators, those weird symbols that we hardly use. So, I guess we are going back to school now.
I’ll try and make this quick so you don’t fall asleep on me. First, Python operators help us make true or false results to use in our code to make decisions. However, you are here to find out what operators are and how to use them.
This topic is part of our Introduction to Python Course. (It’s Free). We like to keep things simple and leverage Google’s Colab which allows you to write python right in your browser. You can follow along with our notebook if you would like. If you want more details on this topic you can visit Python.org.
Topics Covered
What is an Operator ?
Operators are used to perform operations on variables and values. Python divides the operators into the following groups of our course. What a coincidence!
Arithmetic Operators
Just as any other programming language, the addition(+), subtraction(-), multiplication(*), and division(/) operators can be used with values. Arithmetic operators are used with values to perform common mathematical operations.
print(1 + 2 - 1 * 2 / 1)
1.0
Python also has symbols we can use to do some math functions for us. Work smart, not hard I always say. The modulo (%) operator, which is a fancy way of saying I will divide the two numbers and give you the remainder.
print(12 % 5)
2
There is also the floor operator(//) which is the opposite of modulo. This will divide the two numbers and give you the highest it can divide. This will keep you from getting a float number when you divide.
print(31 // 2)
15
Then we have the power operator(**). Which gives you the power to get the power of a number. So 2^8 power would be 256, by the way, I cut and pasted the answer from the cell, ha and I used power 4 times too ( power^4 ).
print(2 ** 8)
256
Just like in our String Formatting post you can use the operators to do things with strings, like add two different strings together. I recommend experimenting with the code that I put here for you. Try other operators and use if statements, check out the Python operator’s section, and see the possibilities you can do with operators.
element1 = "Markdown"
element2 = "Colab "
print(f"{element1} in {element2}" + "is " + "used to make this!")
Markdown in Colab is used to make this!
Assignment Operators
Assignment operators tell values what they can be. Does that sound like someone you know? Just kidding, no one can tell you what to be but yourself. So assignment operators are just operators that include an equal sign with them. This lets python know that you are assigning a value to something.
Try them out using numbers too, just showing this one because it adds it to the string.
value = "number"
print(value)
value += "s"
print(value)
number
numbers
Comparison Operators
This one is a no-brainer but will give a definition for learning purposes. Comparison operators compare two values to each other, like in the examples below. Don’t worry I will have a cheat sheet with all the operators and what they do.
print(5 == 7)
print(5 < 7)
False
True
Logical Operators
Have you ever played Minecraft and built anything with Redstone, then you might know all about logic operators(AND, OR, NOT) or another term logic gates. These operators take in two arguments that will return one output (true or false) depending on the comparison of the two arguments.
If apple is red AND banana is yellow, that will come back true or false. If true give me both, if not give me nothing.
apple = "red"
banana = "yellow"
if apple == "blue" and banana == "yellow":
print("I now have an apple and banana!")
else:
print("I didn't get anything, I turned around and cried.")
I didn't get anything, I turned around and cried.
Membership Operators
These operators will be used most if you are handling strings in lists or dictionaries. Not that gym membership that you bought and never used. Now the membership operator is used to check if a value is in another value or not in the value.
Also, you can look at it like this, if bread is on my list then I’m going to get bread. If not then I’m not yhaving any PB&J when I get home.
groceryList = ["Milk","Eggs","BACON","Bread"]
if "bread" in groceryList:
print("Gonna love that PB&J when I get home!")
else:
print("I forgot that bread! (this Bread is different!!!)")
I forgot that bread! (this Bread is different!!!)
Python Cheat Sheet to Help Code Faster
Operators
Operators are used to perform operations on variables and values. Add, Compare, Check in, ect…
Common Mistakes
You’re not trying to play with code. We know that sometimes you just want answers and that should come after you have tried for a few hours.
Can’t use operators on strings to numbers and numbers to strings.
Lowercase and Uppercase are different values with strings and this “10” is not a number, it is a string.
Operation | Name | Example |
---|---|---|
+ | Addition | i + f |
– | Subtraction | y – o |
* | Multiplication | u * n |
/ | Division | o / t |
% | Modulus | i % c |
** | Exponentiation | e ** t |
// | Floor division | h // i |
== | Equal | s == l |
!= | Not equal | e != a |
> | Greater than | v > e |
< | Less than | a < c |
>= | Greater than or equal to | o >= m |
<= | Less than or equal to | m <= e |
and | Returns True if both statements are true | n < 1 and t < 2 |
or | Returns True if one of the statements is true | p < 1 or l < 2 |
not | Returns False if the result is true | not(e < 1 and a < 2) |
in | Returns True if value is present in the element | s in e |
not in | Returns True if value is not present in the element | t not in y |
Operator | Example | Same As |
– | – | – |
= | a = 1 | a = 1 |
+= | a += 2 | a = a + 2 |
-= | a -= 3 | a = a – 3 |
*= | a *= 4 | a = a * 4 |
/= | a /= 5 | a = a / 5 |
%= | a %= 6 | a = a % 6 |
//= | a //= 7 | a = a // 7 |
**= | a **= 8 | a = a ** 8 |
&= | a &= 9 | a = a & 9 |
|= | a |= 10 | a = a | 10 |
^= | a ^= 11 | a = a ^ 11 |
>>= | a >>= 12 | a = a >> 12 |
\<<= | a \<<= 13 | a = a \<< 13 |
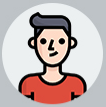
I have my Google IT Support Professional Certificate, and Google IT Automation with Python Specialization Certificate. I continue to develop my skills in networking, operating systems, system administration, troubleshooting, communication, security, and programming. I train constantly through computer courses that include 80% hands-on practice. I love learning new technical skills, analytical skills, and programming through Udemy, Coursera, and any other sources every day. I have 10 years of computer skills and 2 years of programming with Python, C#, JavaScript, and HTML.