Python if else statements are the bread and butter of scripting. The ability of a program to alter its code sequence is called branching, and it’s a key component in making your scripts useful. Python if else statements allow you to create branching for your script, this instructs your computer to make decisions based on inputs.
We as people make decisions every day that change depending on our input like, if I’m broke I’ll try and make some money blogging. We will also go over the conditionals that are used with the Python if else statements.
This topic is part of our Introduction to Python Course. (It’s Free). We like to keep things simple and leverage Google’s Colab which allows you to write python right in your browser. You can follow along with our notebook if you would like. If you want more details on this topic you can visit Python.org.
Topics Covered
What is an if statement?
If you knew that, you wouldn’t be reading this. So, an if statement is used to evaluate a comparison using conditionals. We start with the if keyword, followed by our comparison, then with a colon. The body of the if statement is then indented to the right. If the comparison is True, the code in the body is executed. If the comparison evaluates to False, then the code block is skipped and will move on to the next line of code.
Python If Statement and Conditions
Python uses the logic conditions from mathematics:
- Equal To: a == b
- Not Equal: a != b
- Less Than: a < b
- Less Than or Equal To: a <= b
- Greater Than: a > b
- Greater Than or Equal To: a >= b
These conditions can be used in several ways, most commonly in if statements and loops. In this example, my wishbankaccount
is greater >
than mybankaccount
so it prints "I am BROKE!"
mybankaccount = 0
wishbankaccount = 200
if wishbankaccount > mybankaccount:
print("I am BROKE!!!")
I am BROKE!!!
Indentation
Python uses indentation (empty space at the beginning of a line) to define which body of code goes which line in the code. Here I don’t indent the body of the if statement so Python doesn’t know it goes to the if statement above and gives an IndentationError:
.
mybankaccount = 0
wishbankaccount = 200
if wishbankaccount > mybankaccount:
print("I am BROKE!!!")
File "", line 4
print("I am BROKE!!!")
^
IndentationError: expected an indented block
Elif
The elif keyword is pythons way of saying, if the previous condition is not true, then try this condition. So wishbankaccount
is not >
greater than mybankaccount
which makes it False. Though mybankaccount
is ==
equal to wishbankaccount
so the condition is True and prints "I broke even!"
.
mybankaccount = 200
wishbankaccount = 200
if wishbankaccount > mybankaccount:
print("I am BROKE!!!")
elif mybankaccount == wishbankaccount:
print("I broke even!")
I broke even!
Else
The else keyword is for anything that isn’t caught by the preceding conditions. Like if nothing else is True, do this instead. The top two conditions are False so it prints "I am not broke!"
.
mybankaccount = 300
wishbankaccount = 200
if wishbankaccount > mybankaccount:
print("I am BROKE!!!")
elif mybankaccount == wishbankaccount:
print("I broke even!")
else:
print("I am not broke!")
I am not broke!
Short Hand If and If Else
If you have only one statement to execute, you can put it on the same line as the if statement, or if you have only one if statement, and one else statement, you can put it all on the same line. Make sure with the if else shorthand the body goes first then the if condition, then else condition with body. Here indention is not needed, though this can get lengthy and hard for others to read your code.
mybankaccount = "$2"
wishbankaccount = "$330"
if mybankaccount < wishbankaccount: print(f"mybankaccount = {mybankaccount}")
print(f"mybankaccount = {mybankaccount}") if mybankaccount > wishbankaccount else print(f"wishbankaccount = {wishbankaccount}")
mybankaccount = $2
wishbankaccount = $330
Cheat Sheet Python If Else Statement
Friendly link to Cheat Sheet Page
If Elif Else Cheat Sheet
The python if elif else statement is used to conditionally execute a statement or a block of statements. Conditions can be true or false. They execute one thing when the condition is true and something else when the condition is false.
Comparison operators
- Equal To: a == b
- Not Equal: a != b
- Less Than: a < b
- Less Than or Equal To: a <= b
- Greater Than: a > b
- Greater Than or Equal To: a >= b
Logical operators
- a and b: True if both a and b are True. False otherwise.
- a or b: True if either a or b or both are True. False if both are False.
- not a: True if a is False, False if a is True.
Branching blocks
In Python, we branch our code using if, elif, and else. This is the branching syntax:
if condition1:
body
elif condition2:
body
else:
body
Common Mistakes
An elif
statement must follow an if
statement, and the body will only be executed if the if
statement condition is False. The if
body will be executed if condition1 is True. Now the elif
body will be executed if condition1 is False and condition2 is True. And the else
body will be executed when all the other specified conditions are False.
Typical use
Python if else statements allow you to create branching for your script, this instructs your computer to make decisions based on inputs.
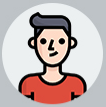
I have my Google IT Support Professional Certificate, and Google IT Automation with Python Specialization Certificate. I continue to develop my skills in networking, operating systems, system administration, troubleshooting, communication, security, and programming. I train constantly through computer courses that include 80% hands-on practice. I love learning new technical skills, analytical skills, and programming through Udemy, Coursera, and any other sources every day. I have 10 years of computer skills and 2 years of programming with Python, C#, JavaScript, and HTML.