This beginner tutorial will teach you about the Python While Loop. They can be one of your most commonly used functions, but can sometimes drive you loopy. Your Journey Into Python will show you how to create different while loops and common problems when creating while loops.
This topic is part of our Introduction to Python Course. (It’s Free). We like to keep things simple and leverage Google’s Colab which allows you to write python right in your browser. You can follow along with our notebook if you would like. If you want more details on this topic you can visit Python.org.
Topics Covered
What is a Python While Loop?
A while loop will continuously execute code depending on the value of a condition. It begins with the keyword while, followed by a comparison to be evaluated, then a colon. On the next line is the code body to be executed, indented to the right
t. The code in the body will only be executed if the comparison is evaluated to be true. What sets a while loop apart, however, is that this code block will keep executing as long as the evaluation statement is true. Once the statement is no longer true, the loop exits, and the next line of code will be executed.
The while Loop
The while function instructs your computer to continuously execute your code based on the value of a condition:
i = 1
while i <= 4:
print(i)
i += 1
1
2
3
4
The else Statement
With the else statement we can run a block of code once when the condition is no longer true:
i = 1
while i <= 4:
print(i)
i += 1
else:
print("i is no longer less than 4")
1
2
3
4
i is no longer less than 4
The break Statement
With the break statement we can stop the loop even if the while condition is true:
i = 1
while i != 100:
print(i)
if i == 3:
print('i stopped at 3')
break
i += 1
1
2
3
i stopped at 3
The continue Statement
With the continue statement we can stop the current iteration, and continue with the next:
i = 0
while i < 5:
i += 1
if i == 3:
print("We skipped 3")
continue
print(i)
1
2
We skipped 3
4
5
Why Initializing Variables Matters
You’ll want to watch out for the most common mistake of forgetting to initialize variables. If you try to use a variable without first initializing it, you’ll run into a NameError:
. Another common mistake to watch out for is forgetting to initialize variables with the correct value or having the variable change unintentionally in the while loop.
while i < 4:
i += 1
if i == 3:
continue
print(i)
This will run, but you will not get any results because the initialized variable is missing. For more info check out Python.org, if you haven’t already.
While Loops Cheat Sheet
Printer friendly link to Cheat Sheet
While Loops
A while loop executes the body of the loop while the condition remains True.
Syntax
while condition:
body
Common mistakes
Failure to initialize variables. Make sure all the variables used in the loop’s condition are initialized before the loop.
Unintended infinite loops. Make sure that the body of the loop modifies the variables used in the condition so that the loop will eventually end for all possible values of the variables.
Typical use
While loops are mostly used when there’s an unknown number of operations to be performed, and a condition needs to be checked at each iteration.
Break & Continue
You can interrupt the while loop using the break keyword. We normally do this to interrupt a cycle due to a separate condition.
You can use the continue keyword to skip the current iteration and continue with the next one. This is typically used to jump ahead when some of the elements of the sequence aren’t relevant.
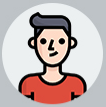
I have my Google IT Support Professional Certificate, and Google IT Automation with Python Specialization Certificate. I continue to develop my skills in networking, operating systems, system administration, troubleshooting, communication, security, and programming. I train constantly through computer courses that include 80% hands-on practice. I love learning new technical skills, analytical skills, and programming through Udemy, Coursera, and any other sources every day. I have 10 years of computer skills and 2 years of programming with Python, C#, JavaScript, and HTML.