In our Journey Into Python for beginners, we are going to learn about Python Dictionaries with Nested Dictionary. In addition, you will learn how to iterate over them to get the keys and values.
Altogether learning dictionaries is going to help you understand the fundamentals. In addition, you going to learn how to retrieve and store data to use anywhere. For instance, dictionaries don’t allow you to have duplicate keys, but the value of keys can be the same. Similarly, Python has another data type for Python called lists.
This topic is part of our Introduction to Python Course (It’s Free). We like to keep things simple and leverage Google’s Colab which allows you to write python right in your browser. You can follow along with our notebook if you would like and if you want more details on this topic you can visit Python.org.
Topics Covered
What is a Python Dictionary?
A Python dictionary is a collection of key-value pairs, each key-value pair combines the key to its value. Most importantly, when creating a dictionary we use curly brackets to begin, then between the key and the value, we use a colon and separate each pair by commas.
Furthermore, the keys and values in a dictionary can be strings, integers, floats, tuples, and more. As a rule Python dictionaries can only have one key or any new key will overwrite the old key.
Creating a Dictionary
In the same way, a Python Dictionary is like an English dictionary. Where the word would be the key and the definition would be the value. Let’s check out the example below.
Creating an empty dictionary
In this example, it is as simple as naming your variable and giving it an empty dictionary using curly brackets.
example = {}
Creating your first dictionary with data
To demonstrate this, we will first put the data into the new dictionary. You will notice that when you do this, we use strings inside the curly brackets. The first string will be the key which has a colon after it. Next, we will add another string, this is the value of the key. We will then print the variable that that has the dictionary key-value pair.
example = {'word': "definition"}
print(example)
{'word': 'definition'}
Using the type function
In this example, we use the build-in type function to check what type of variable we have.
print(type(example))
<class 'dict'>
Types of Keys and Values
Dictionaries can have different kinds of variable types that they can use for keys or key values. First is the string type, which can be both key and value. Next is integers which can also be both. Since integers can be both, so can float integers.
Likewise, you can put tuples in dictionaries as both key and value, but using tuples for keys may not be very useful. Now alternatively lists can be in dictionaries but only for values of the keys.
Strings
user = {'first_name': 'Jack', 'last_name': 'Benimble'}
print(user)
{'first_name': 'Jack', 'last_name': 'Benimble'}
Integers and Floats
int_floats = {1: 1, 2: 2.5, 3: 3.75, 2.50: 5}
print(int_floats)
{1: 1, 2: 2.5, 3: 3.75, 2.5: 5}
Tuples
lunch = {('main1', 'main2'): ('hotdog', 'bun'), ('side1', 'side2'): ('fries', 'drink')}
print(lunch)
{('main1', 'main2'): ('hotdog', 'bun'), ('side1', 'side2'): ('fries', 'drink')}
List
book = {'chapters': ([1, 2]), 'sections': ([1.1, 1.2, 2.1, 2.2])}
print(book)
for key, value in book.items():
print(key, value)
{'chapters': [1, 2], 'sections': [1.1, 1.2, 2.1, 2.2]}
chapters [1, 2]
sections [1.1, 1.2, 2.1, 2.2]
Leverage dictionaries
In addition to the different types of data that can go into a dictionary. There is the ability to alter the contents of a created dictionary. In this section, you will first learn about storing data, just like when we created that variable.
You can start off with an empty dictionary or you can create it with your own values. Admittedly this sounds simple but when you start working with large amounts of data this can get crazy. Obviously, you want to take existing dictionaries and add that to your own dictionary.
So here are the examples of storing, adding, updating, and deleting.
Storing, Adding, Updating, Deleting Data
test_dict = {}
print(type(test_dict))
test_dict = {'key1': 'value1'}
print(test_dict)
test_dict['key2'] = 'value2'
test_dict2 = {'key3': 'value3'}
test_dict.update(test_dict2)
print(test_dict)
test_dict.pop('key3')
print(test_dict)
<class 'dict'="">
{'key1': 'value1'}
{'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
{'key1': 'value1', 'key2': 'value2'}
List to Dictionaries
first_names = ['Jack', 'Mary', 'John']
last_names = ['Benimble', 'Jane', 'Doe']
users = dict(zip(first_names, last_names))
print(users)
{'Jack': 'Benimble', 'Mary': 'Jane', 'John': 'Doe'}
Iterating Over Dictionaries With Lists For Values
book = {'chapters': ([1, 2]),
'sections': ([1.1, 1.2, 2.1, 2.2]),
'sub_sections': (['1.1a', '1.1b', '1.2a', '1.2b', '2.1a', '2.1b', '2.2a', '2.2b'])}
for key, values in book.items():
print(key)
if isinstance(values, list):
for value in values:
print(value)
else:
print(value)
chapters
1
2
sections
1.1
1.2
2.1
2.2
sub_sections
1.1a
1.1b
1.2a
1.2b
2.1a
2.1b
2.2a
2.2b
Iterating Over Dictionaries
for key, value in users.items():
print(key,value)
Jack Benimble
Mary Jane
John Doe
Nested Dictionaries
nested = {'dictA': {'key1': 'value1',
'key2': 'value2'},
'dictB': {'key3': 'value3',
'key4': 'value4'}
}
nested['dictC'] = ({'key5': 'value5', 'key6': 'value6'})
print(nested)
{'dictA': {'key1': 'value1', 'key2': 'value2'}, 'dictB': {'key3': 'value3', 'key4': 'value4'}, 'dictC': {'key5': 'value5', 'key6': 'value6'}}
Iterating Over Nested Dictionaries
def nested_dict_iterator(nested_dict):
for key, value in nested_dict.items():
if isinstance(value, dict):
for pair in nested_dict_iterator(value):
yield(key, *pair)
else:
yield(key, value)
for pair in nested_dict_iterator(nested):
print(pair)
('dictA', {'key1': 'value1', 'key2': 'value2'})
('dictB', {'key3': 'value3', 'key4': 'value4'})
('dictC', {'key5': 'value5', 'key6': 'value6'})
Dictionary Methods Cheat Sheet
Definition
x = {key1:value1, key2:value2}
Operations
- len(dictionary) – Returns the number of items in the dictionary
- for key in dictionary – Iterates over each key in the dictionary
- for key, value in dictionary.items() – Iterates over each key,value pair in the dictionary
- if key in dictionary – Checks whether the key is in the dictionary
- dictionary[key] – Accesses the item with key key of the dictionary
- dictionary[key] = value – Sets the value associated with key
- del dictionary[key] – Removes the item with key key from the dictionary
Methods
- dict.get(key, default) – Returns the element corresponding to key, or default if it’s not present
- dict.keys() – Returns a sequence containing the keys in the dictionary
- dict.values() – Returns a sequence containing the values in the dictionary
- dict.update(other_dictionary) – Updates the dictionary with the items coming from the other dictionary. Existing entries will be replaced; new entries will be added.
- dict.clear() – Removes all the items of the dictionary
Check out the official documentation for dictionaries at Python.org.
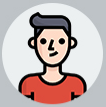
I have my Google IT Support Professional Certificate, and Google IT Automation with Python Specialization Certificate. I continue to develop my skills in networking, operating systems, system administration, troubleshooting, communication, security, and programming. I train constantly through computer courses that include 80% hands-on practice. I love learning new technical skills, analytical skills, and programming through Udemy, Coursera, and any other sources every day. I have 10 years of computer skills and 2 years of programming with Python, C#, JavaScript, and HTML.