In this post, we will learn how to use and create Python modules. Python Modules are a large part of programming by allowing developers to share their work with others. Not to mention that someone out there has run into a problem while coding and has come up with a solution.
So when you are writing your own code, you can import other people’s solutions. Python has an extensive community of programmers that like to share their code. Altogether, in my opinion, Python is the most user-friendly and most supportive community.
Now for this post! I will be using Colab for all my coding. You can use the provided examples in any IDE for Python. Alternatively, I will have code snippets for those that want to use Colab. So let us begin your journey into Python Modules.
This topic is part of our Introduction to Python Course. (It’s Free). We like to keep things simple and leverage Google’s Colab which allows you to write python right in your browser. You can follow along with our notebook if you would like. If you want more details on this topic you can visit Python.org.
Topics Covered
What are Python Modules?
Python Modules can be referred to as code library files. That file contains classes and/or functions external scripts can access and use.
If you would like to know more about functions and classes. You can find out at these courses. Python Functions and Python Classes
For example, you can have a module that handles the payment of your employees. Then have a module that keeps track of your current employees. You can then create a script that uses both modules to generate a report of each employee’s pay. This allows you to alter those modules without affecting the original Python Modules.
Create a Module and import it
Now that you understand what a module is and what it does. Let me give you an example below. As a rule, your module will need to be saved as a .py file. Python is looking through directories for that type of file format.
In the case of my example below, I will use Colab to download my code as a .py file. Although you can use any IDE for Python to save it as a .py file. Make sure that you save it in the directory that you are working in.
Another thing you can do is use import sys
and print(sys.path)
to see what directories Python is looking in.
# Save as your_module.py
print("Module imported successfully!")
villain = {
"firstName":"Freddy",
"lastName":"Krueger",
"knownFor":"Terrorizing kids in their dreams."
}
def print_function():
print("You Horror Movie Villain is:")
print(f"{firstName} {lastName}")
print(f"Best Known For: {knownFor}")
By this point, you should have a file saved in your directory as a .py file. In the next two examples, I show you how to set up Colab. This is so Colab can use your google drive to import your modules. I am saving my module in MyDrive to use Colab to import.
The code below will mount my drive to Colab and change the file path to MyDrive. You will want to run these cells if you are following along in Colab.
# For Colab to get access to your google drive
from google.colab import drive
drive.mount("/content/drive",force_remount=True )
Mounted at /content/drive
# This will change the file path
import sys
sys.path.insert(0,"/content/drive/MyDrive")
Let’s import our module. You can see that as soon as we import, it prints something. This is because the module ran once as soon as it was imported, now keep that in mind. When the module runs, anything not in a function or class will execute.
You have now created your first module and imported it into your script. If you get an error message, make sure you check the file path and the spelling. If you’re in Colab, sometimes you have to restart the runtime and then rerun the cells.
import your_module
Module imported successfully!
How to access functions and variables
If everything went correctly in the last example. We have imported our first module into our script. Now we want to access the variable that is inside the module. This is done by typing `your_module.villain`. This can also be followed by a key to give its value.
print(your_module.villain)
print(your_module.villain["firstName"])
{'firstName': 'Freddy', 'lastName': 'Krueger', 'knownFor': 'Terrorizing kids in their dreams.'}
Freddy
Using modules helps clean up your code when working on complex projects. You can break up your code into smaller, more manageable pieces of code. When you create modules, the code should be functions or classes.
To access the functions in a module is similar to the variable. You type `your_module.print_function()` and the code inside the function will run.
your_module.print_function()
You Horror Movie Villain is:
Freddy Krueger
Best Known For: Terrorizing kids in their dreams.
Learn different ways to import Modules
Alright, so we know how to create a module. We now have to import your module and access the contents. Though what if you want to import some of the content? Well, Python lets you do that too in different ways.
First, I would like to go over the module import. When you import a module, you can change the name to whatever you want. You can do this by importing the module as the name of your choice, like the example below.
import your_module as ym
Now I will go over how you can import certain content from your module. First, you can type `from your_module import print_function` to import just that function. Remember that you can not access that villain variable in the module now. Additionally, you can import functions and call them what you want.
Another way you can import functions is with the asterisk after the import. This will allow access to all the content, but this is rarely used and frowned upon. The examples below will show what each call will look like.
from your_module import print_function
from your_module import print_function as pf
from your_module import*
Getting info on built-in Modules
Anytime you use Python, you have access to a wide range of modules. These modules can range from creating graphics to solving complex math formulas. You can find a list of built-in modules at Python’s Standard Library.
I will talk about how to find out what functions they have and what they do once you have found a module that might help you with your script. You can use the `dir(module)` function to get a list of the usable functions in a module.
Additionally, once you find a function that you want. You can use the `help(module.function)` function to get additional information on the function. Below are some examples of a module using the dir() and help function.
print(dir(your_module))
print(help(your_module.villain))
['__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', 'print_function', 'villain']
Help on dict object:
class dict(object)
| dict() -> new empty dictionary
| dict(mapping) -> new dictionary initialized from a mapping object's
| (key, value) pairs
| dict(iterable) -> new dictionary initialized as if via:
| d = {}
| for k, v in iterable:
| d[k] = v
| dict(**kwargs) -> new dictionary initialized with the name=value pairs
| in the keyword argument list. For example: dict(one=1, two=2)
ect...
A little on Packages
Packages are a namespace that includes other packages and modules. They are just like directories on your computer with some differences. Every package in Python is a directory that must include a unique file referred to as an __init__.py. This file can be empty, showing Python that this is a directory of a Python package.
This means that you can import it just like any other module. Below is a minimum of what a Python package must include.
main_directory_folder
__init__.py
# This file is required
# File can be empty
your_module.py
# Has functions to use
import main_directory_folder.your_module
from main_directory_folder import your_module
Cheat Sheet Python Modules:
Python Modules can be referred to as code library files. That file contains classes and/or functions that external scripts can use.
Tips
- Module has to be a save in .py file format
- Python needs to know where to look for your module
- Use sys.path to find where Python is looking for module
- Use dir(module) and help(module.function) for module information
Functions
- Python’s system management module – import sys –
- Displays the paths that Python looks – for modules sys.path –
- How to import modules – import module –
- Rename the module – import module as ym –
- Import just one function of module – from module import function –
- Rename the function – from module import function as pf –
- Used to import all functions of module – from module import* –
Common Mistakes
Incorrect PYTHONPATH for module
Forgetting your period between the module name and function name
Typical Use
Modules separate complex scripts into manageable groups with similar functions. This will also clean up your code and be more reader-friendly.
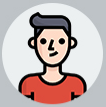
I have my Google IT Support Professional Certificate, and Google IT Automation with Python Specialization Certificate. I continue to develop my skills in networking, operating systems, system administration, troubleshooting, communication, security, and programming. I train constantly through computer courses that include 80% hands-on practice. I love learning new technical skills, analytical skills, and programming through Udemy, Coursera, and any other sources every day. I have 10 years of computer skills and 2 years of programming with Python, C#, JavaScript, and HTML.