In today’s ‘Journey Into Python’ we will explore the for loop. Now there are two types of loops in Python, for loops and while loops. There is a lot you can do with loops and is very useful in iterating over data.
You can go through a list of files and check what type of file they are. This can go even further by looping through the files to find and change their type. As you can see the possibilities are only limited by you.
This topic is part of our Introduction to Python Course. (It’s Free). We like to keep things simple and leverage Google’s Colab which allows you to write python right in your browser. You can follow along with our notebook if you would like. If you want more details on this topic you can visit Python.org.
Topics Covered
What is a for loop?
A for loop is used for iterating over a sequence (that is either a list, a tuple, a dictionary, a set, or a string). With the for loop, we can execute a set of statements, once for each item in a list, tuple, set, etc. More details on the for loop can be found at the source Python.org.
Checking out the Range() Function
The range() function can take one, two, or three parameters:
One parameter will create a sequence, one-by-one, from zero to one less than the parameter.
Two parameters will create a sequence, one-by-one, from the first parameter to one less than the second parameter.
Three parameters will create a sequence starting with the first parameter and stopping before the second parameter, but this time increasing each step by the third parameter.
for i in range(6):
print(i)
0
1
2
3
4
5
for i in range(1,6):
print(i)
1
2
3
4
5
for i in range(2,11,2):
print(i)
2
4
6
8
10
Looping Through a String
When looping through a string keep in mind that a for loop has to have a sequence. With the example below it automatically goes through each letter because it can’t iterate a single element.
string = 'string'
for i in string:
print(i)
s
t
r
i
n
g
This example loops through a tuple, a list will do the equivalent and a dictionary will print just the keys.
loop = ('loop','de','loop')
for i in loop:
print(i)
loop
de
loop
The break Statement
You can interrupt the for loop using the break keyword. We normally do this to interrupt a cycle due to a separate condition.
Here the break Statement is after the print() function
fruits = ["apple", "banana", "cherry"]
for x in fruits:
print(x)
if x == "banana":
print("Hey, where's my cherry!!!")
print("I'm gonna wreck it!")
break
apple
banana
Hey, where's my cherry!!!
I'm gonna wreck it!
Here the break Statement is before the print() function
fruits = ["apple", "banana", "cherry"]
for x in fruits:
if x == "banana":
print("I ate the banana and cherry!!!")
break
print(x)
apple
I ate the banana and cherry!!!
The Continue Statement
You can use the continue keyword to skip the current iteration and continue with the next one. This is typically used to jump ahead when some of the elements of the sequence aren’t relevant.
fruits = ["apple", "banana", "corn", "cherry"]
for x in fruits:
if x == "corn":
print("There's a vegtable in our fruit basket!!!")
continue
print(x)
apple
banana
There's a vegetable in our fruit basket!!!
cherry
Nested for Loops
Here we have a nested for loop that prints a set of numbers 0 through 6, but we don’t want to print the same number twice. So the way this works is every time the value of the left for loop changes, the range value in the right for loop changes.
We also use a parameter called end, which instead of creating a new line it adds the value of end and continues to the right.
for left in range(7):
for right in range(left, 7):
print(f'[{left}|{right}]', end=" ")
print()
[0|0] [0|1] [0|2] [0|3] [0|4] [0|5] [0|6]
[1|1] [1|2] [1|3] [1|4] [1|5] [1|6]
[2|2] [2|3] [2|4] [2|5] [2|6]
[3|3] [3|4] [3|5] [3|6]
[4|4] [4|5] [4|6]
[5|5] [5|6]
[6|6]
Let’s say we have a few mammals in a big cage and we want a list of who will eat who. We know the mammal won’t eat itself, so we take the list and loop through the mammals. Then we loop through it again looking for itself and if it doesn’t equal itself print the list.
oz = ['Lions', 'Tigers', 'Bears', 'Humans']
for mammals in oz:
for itself in oz:
if mammals != itself:
print(f'{mammals} will eat {itself}')
Lions will eat Tigers
Lions will eat Bears
Lions will eat Humans
Tigers will eat Lions
Tigers will eat Bears
Tigers will eat Humans
Bears will eat Lions
Bears will eat Tigers
Bears will eat Humans
Humans will eat Lions
Humans will eat Tigers
Humans will eat Bears
Common mistakes new developers make:
The most common mistake with for loops is forgetting they iterate over sequences. Using a single sequence the interpreter will refuse to iterate over the element. So if we tried for x in 10 print(x)
, this would try to iterate over the single element of the integer 10, Python will give a TypeError: integers are not iterable
.
There are two ways around this issue, depending on what you’re trying to do. If you want to go from 0 to 10, then you need to use the range()
function, so for x in range(11) print(x)
, but if you’re trying to iterate over a list that has integer 10 as the only element, then you need to write it between square brackets, for x in list[10] print(x)
.
Cheat Sheet Python For Loop
Friendly link to Cheat Sheet Page
For Loops
A for loop iterates over a sequence of elements, executing the body of the loop for each element in the sequence.
Syntax
for 'variable' in 'sequence':
body
The range() function
- range() generates a sequence of integer numbers. It can take one, two, or three parameters:
- range(n): 0, 1, 2, … n less 1
- range(x,y): x, x+1, x+2, … y less 1
- range(p,q,r): p, p+r, p+2r, p+3r, … q less 1 (if it’s a valid increment)
Common Mistakes
Forgetting that the upper limit of a range() isn’t included.
Iterating over non-sequences. Integer numbers aren’t iterable. Strings are iterable letter by letter, but that might not be what you want.
Typical use
For loops are mostly used when there’s a pre-defined sequence or range of numbers to iterate.
Break & Continue
You can interrupt the for loop using the break keyword. We normally do this to interrupt a cycle due to a separate condition.
You can use the continue keyword to skip the current iteration and continue with the next one. This is typically used to jump ahead when some of the elements of the sequence aren’t relevant.
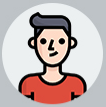
I have my Google IT Support Professional Certificate, and Google IT Automation with Python Specialization Certificate. I continue to develop my skills in networking, operating systems, system administration, troubleshooting, communication, security, and programming. I train constantly through computer courses that include 80% hands-on practice. I love learning new technical skills, analytical skills, and programming through Udemy, Coursera, and any other sources every day. I have 10 years of computer skills and 2 years of programming with Python, C#, JavaScript, and HTML.